What is Unit Testing? What are JUnit tests in Java?
What is Unit Testing? What are JUnit tests in Java? This is what nowadays we are hearing more. Here is an small details about the same.
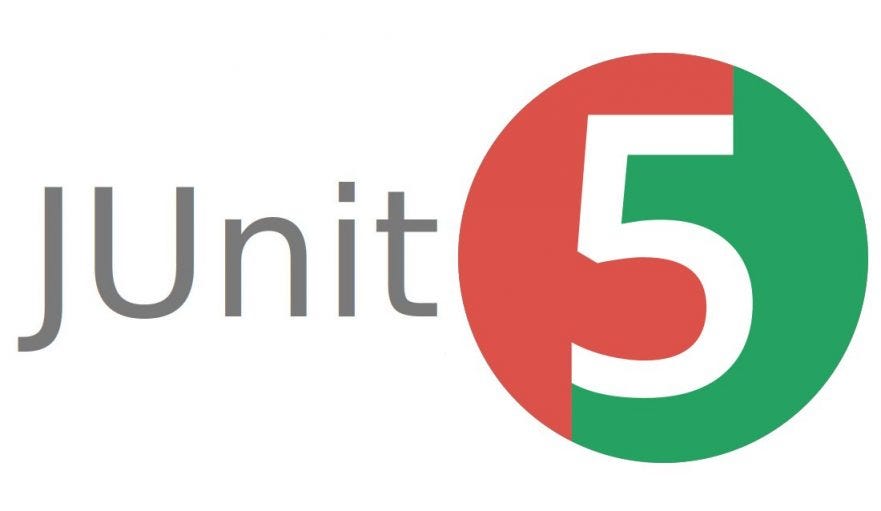
Joshua Bloch who is the author of Effective Java once said, “Unit tests are essential! If you don’t have them, you don’t know whether your code works. Having a good set of unit tests gives you much more confidence that your code works in the first place. And that you don’t introduce bugs as you maintain them. Inevitably, you will introduce bugs, but your unit tests will often let you find the bugs as soon as you introduce them. So you can fix them before they cause any damage”. He answered this to a question asked “Why engineers don’t write unit tests to the code?”
Link to complete interview – https://www.oracle.com/technical-resources/articles/javase/bloch-effective-08-qa.html
However this brings to answer our first question, “What is unit testing?”
Unit testing is a technique to test each and every block of code as an individual entity to ensure its logic and correctness.
Unit testing is very essential to set standards and assurance the code is being logically correct. It also ensures each individual unit works as intended.
How to achieve it using the JUnit framework?
However, there are so many frameworks available to achieve unit testing where the JUnit being very popular in Java Universe.
We will discuss few examples how unit tests are written in Java.
public class CalculateArea(){
public long areaOfSquare(int side){
return side*side;
}
public double area of circle(int radius){
return 3.14 * radius * radius;
}
}
The above methods we are trying calculate area of square and circle.
Lets try to test them with JUnit,
First lets add the required dependency to pom.xml
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<scope>test</scope>
</dependency>
@Test
public void squareareaTest() {
CalculateArea test = new CalculateArea();
assertEquals(0, test.areasquare(0));
}
Now if you observe the method we have an annotation @Test, this marks the test to be a Unit test. Assert statement is used to test the values against the known value and the value returned from the method.
assertequals(Expected, actual);
The tests pass only when the expected value match with the actual value, hence making sure the logic of our implementation is meeting the expectation.
Key points to remember :
- JUnit is just a proof of confidence that our code is logically correct.
- Also on that is only one of the measure and not the only measure to find correctness.
- JUnits helps to track and tackle the bugs introduced during enhancements and fix them then and there.
- It is a good practice to unit test the code how ever not madatory.
- On a shocking note codes of many open source libraries are poorly tested.
Unit Testing JUnit tests
And also if you required any technology you want to learn, let us know below we will publish them in our site http://tossolution.com/
Like our page in Facebook and follow us for New technical information.
Unit Testing JUnit tests
Hey there! Quick question that’s completely off topic.
Do you know how to make your site mobile friendly? My weblog looks weird when viewing from my
iphone. I’m trying to find a template or plugin that might be able to fix this problem.
If you have any suggestions, please share. Many thanks!