What is NULL? What is a Null pointer exception(NPE)? How to deal with it?
Let’s begin with a basic definition of matter, anything that occupies space and has mass is called matter. Similarly what is that doesn’t have mass and doesn’t occupy space? (The answer is not antimatter) Yes, there exists such phenomena. What is NULL? What is a Null pointer exception(NPE)? How to deal with it?
What is NULL?
Now coming to the world of programming let us define what is “NOT NULL”? Anything that occupies memory and has an address is not null. On the contrary, NULL doesn’t exist in memory and doesn’t have any address. Let us discuss it with an example of a class.
Class Car{
String name;
String make;
int year;
}
Now we have the definition of Car class, suppose if we declare the car using new keyword,
Car maruthi = new Car();
Here we are declaring an instance(Object) of car, which means the car object has address and occupies memory. If we do a System.out.print it will display the address of the object.
System.out.println(maruthi);
com.deekshith.bennur.Car&@356768
You might have seen output like these, it clearly says Maruthi – the car instance is not null.
What is Null Pointer Exception?
Then what is null in the given instance of Car, well the underlying fields of car, model, and make are null but integer field year is not null, by default it is set to 0. Because we have defined the values for the instance. Let us discuss with another snippet
1 System.out.println(maruthi.getmake());
2 System.out.println(maruthi.getModel());
When the 1st statement is executed it will print 0 as by default int values are assigned to 0.
When the 2nd statement is executed it is going to print null, but why we have the Maruthi which is not null?
The answer is we never set the value of Model to our instance Maruthi, that is the reason it is null.
How to deal with NPE?
Then what is a Null Pointer Exception and when will it occur? When an operation is performed on a null value a runtime exception occurs that is NPE. An NPE occurs when we are referring to an instance that is null.
Case 1. When we try to retrieve a data from DB and if the data doesn’t exist the DB returns is null when we try to operate on that instance it will throwing the NPE.
Case 2. As we discussed earlier when an operation is performed on an instance where a certain field is null, a null pointer exception is thrown.
How do we when NPE occurs? No, we can’t predict it that’s why it’s a runtime exception. How do we deal with that? The best possible way is by doing a null check.
Car duster = DB.get(where make="Renault");
if(duster!=null){
// perform any operation on instance duster
}
In the above code block you can see we are doing a null check so that we don’t end up with a NPE.
The second method would be surrounding try catch block.
try{
String model = duster.getModel();
}catch(Exception e){
e.printstacktrace();
}
In this even though our instance duster is null it will be caught in the catch block.
What is NULL? What is a Null pointer exception(NPE)? How to deal with it?
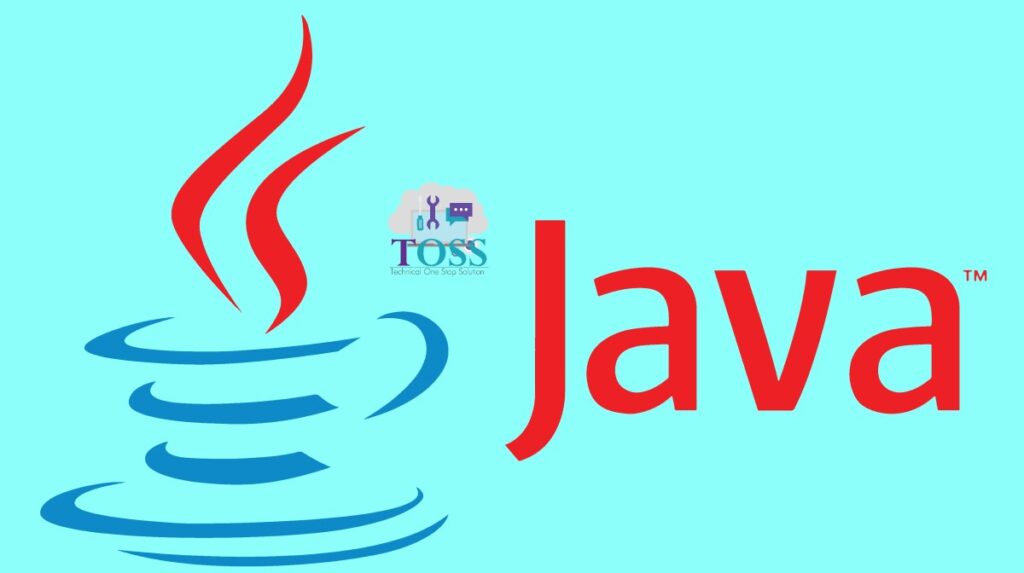
To get more knowledge on Java click here.